Image Rollovers Without Inline HTML Markup
Using non-obtrusive, standards compliant cross-browser code for making image rollovers with ease.
fixed a typo, thanks to Slade for the heads-up - 08.09.2005
created on 08.09.2005
Introduction
Hey there.. I don't know if you are following the trend in Javascripting, but inline javascripts are becoming a thing of the past, hopefully, for a number of good reasons:
- Inline scripts increase HTML size, and they are against the seperation of content from style principle.
- Using lots of inline scripts makes it harder to maintain the code.
- You have to do just too many changes simply for changing a rollover image.
Example Rollovers
Hover these (most basic usage):
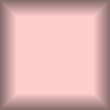
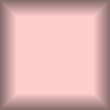
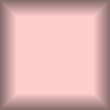
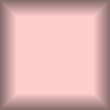
Hover and click these:
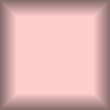
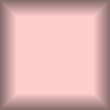
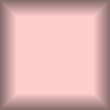
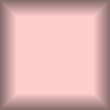
Hover and click these, there is no restore:
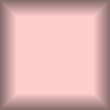
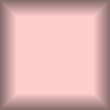
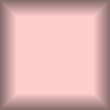
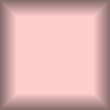
Example Basic Usage
Required HTML
Standard HTML<img src='sampleroll_nm.png' />
Required Javascript
JavascriptprepareImageSwap(document.body);
Required hover image name for this hover:
sampleroll_hv.png
That's it!
This was the most basic usage, it is hover with restore. You just name your images so that they have "_nm" in the end of their name, and our script knows that they are our target images, and replaces the "_nm" with "_hv" on hover, and restores the image on mouse out. The good thing is, none of our code destroys validity of our HTML, so the resulting code is valid HTML/XHTML.
Of course we are assuming you have included the you can check the function used for this page here.)
function in your page either by linking or embedded in the head element of your page, and ensuring you call it after document has loaded (window.onload, you know the drill, butAdvanced Usage
Variables that can be passed to the function
prepareImageSwap(elem, mouseOver, mouseOutRestore, mouseDown, mouseUpRestore, mouseOut, mouseUp)
- Elem:
- Element passed as object or its ID as string
- Element || Element's ID (string)
- mouseOver
- Do image swap on mouse hover?
- true || false (default: true)
- mouseOutRestore
- Restore image on mouse-out?
- true || false (default: true)
- mouseDown
- Do image swap on mouse-down?
- true || false (default: false)
- mouseUpRestore
- Restore image on mouse-button-up?
- true || false (default: false)
- mouseOut
- Use separate image for mouse-out?
- true || false (default: false)
- mouseUp
- Use separate image for mouse-up?
- true || false (default: false)
Naming convention for images
Assuming base image name will be
and our image is a .- foo_nm.jpg
- Our base image name
- foo_hv.jpg
- Image name for hover
- foo_md.jpg
- Image name for mouse-down
- foo_ou.jpg
- Image name for mouse-out (when you want a separate image for mouse-out instead of restoring to original)
- foo_mu.jpg
- Image name for mouse-up (when you want a separate image for mouse-up instead of restoring to original)
The code
Javascriptfunction prepareImageSwap(elem,mouseOver,mouseOutRestore,mouseDown,mouseUpRestore,mouseOut,mouseUp) { //Do not delete these comments. //Non-Obtrusive Image Swap Script V1.1 by Hesido.com //Attribution required on all accounts if (typeof(elem) == 'string') elem = document.getElementById(elem); if (elem == null) return; var regg = /(.*)(_nm\.)([^\.]{3,4})$/ var prel = new Array(), img, imgList, imgsrc, mtchd; imgList = elem.getElementsByTagName('img'); for (var i=0; img = imgList[i]; i++) { if (!img.rolloverSet && img.src.match(regg)) { mtchd = img.src.match(regg); img.hoverSRC = mtchd[1]+'_hv.'+ mtchd[3]; img.outSRC = img.src; if (typeof(mouseOver) != 'undefined') { img.hoverSRC = (mouseOver) ? mtchd[1]+'_hv.'+ mtchd[3] : false; img.outSRC = (mouseOut) ? mtchd[1]+'_ou.'+ mtchd[3] : (mouseOver && mouseOutRestore) ? img.src : false; img.mdownSRC = (mouseDown) ? mtchd[1]+'_md.' + mtchd[3] : false; img.mupSRC = (mouseUp) ? mtchd[1]+'_mu.' + mtchd[3] : (mouseOver && mouseDown && mouseUpRestore) ? img.hoverSRC : (mouseDown && mouseUpRestore) ? img.src : false; } if (img.hoverSRC) {preLoadImg(img.hoverSRC); img.onmouseover = imgHoverSwap;} if (img.outSRC) {preLoadImg(img.outSRC); img.onmouseout = imgOutSwap;} if (img.mdownSRC) {preLoadImg(img.mdownSRC); img.onmousedown = imgMouseDownSwap;} if (img.mupSRC) {preLoadImg(img.mupSRC); img.onmouseup = imgMouseUpSwap;} img.rolloverSet = true; } } function preLoadImg(imgSrc) { prel[prel.length] = new Image(); prel[prel.length-1].src = imgSrc; } } function imgHoverSwap() {this.src = this.hoverSRC;} function imgOutSwap() {this.src = this.outSRC;} function imgMouseDownSwap() {this.src = this.mdownSRC;} function imgMouseUpSwap() {this.src = this.mupSRC;}
The code, explained
Please check back later for this, just subscribe to my feed for updates to Hesido.com.